So, I got this problem the other day where I needed to resize some images, but I wanted them to always scale from the top. You know, like when you resize a window from the bottom, the top stays fixed? That’s what I was going for.
It wasn’t as straightforward as I thought it would be. I mean, normally when you resize stuff, it either scales from the center or from the top-left corner. But I needed something different.
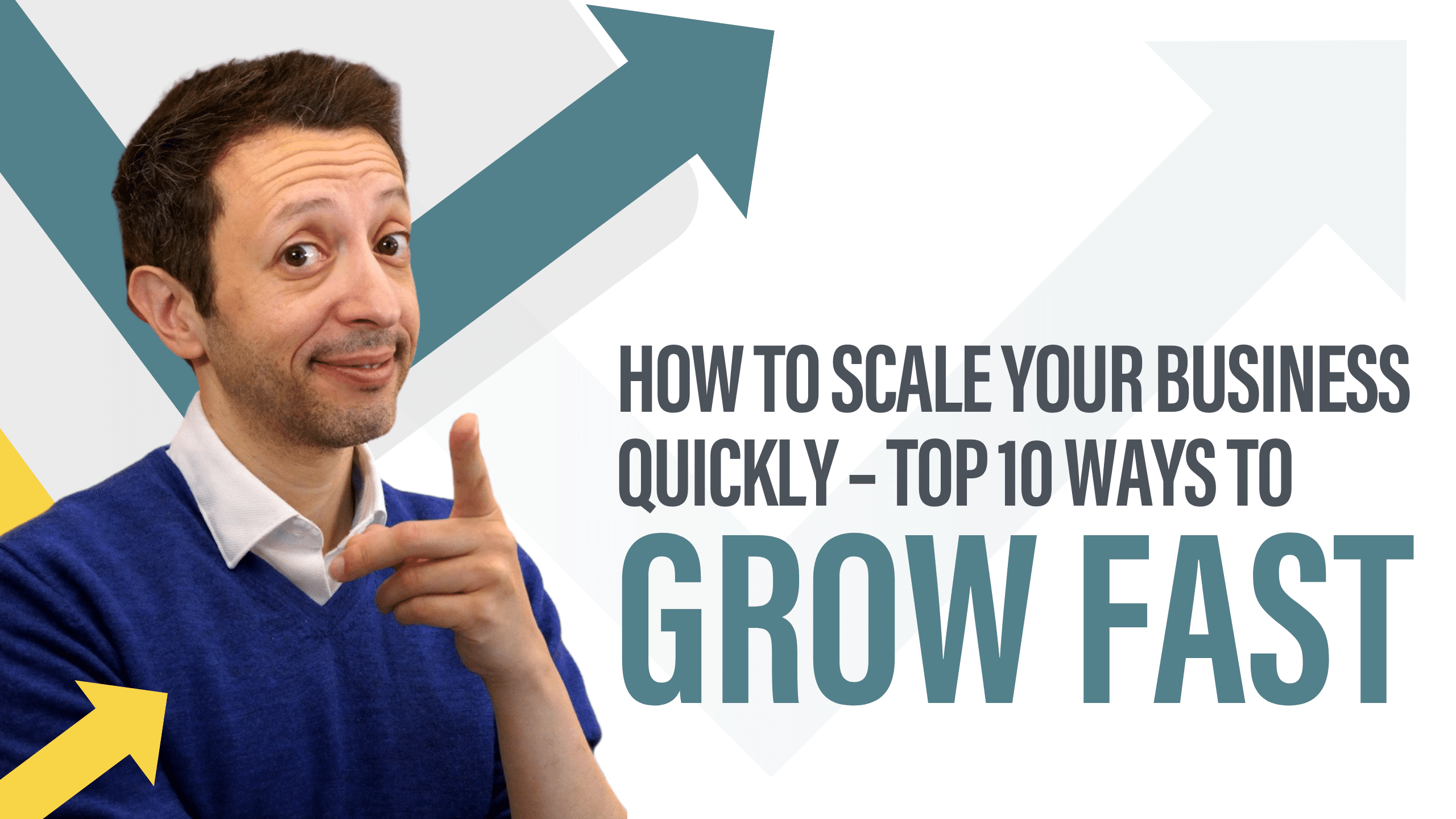
First, I tried messing around with the usual CSS properties. You know, the transform and transform-origin stuff. I set the transform-origin to top, hoping that would do the trick.
Here’s a snippet of what that looked like:
-
Tried to change the origin of scaling.
.my-image {
transform-origin: top;
transform: scale(0.8);
But nope, it scaled from the top, but didn’t keep it fixed, like I had hoped to achieve.
So, I started digging around, reading some articles, and trying out different approaches. Then I remembered something about how positioning works in CSS. And that was when I used relative and absolute positioning.
I wrapped my image in a container and set the container’s position to relative. Then, I set the image’s position to absolute and aligned it to the top of the container.
Here’s how that looked:
-
Created a container for the image.
.image-container {
position: relative;
width: 300px;
height: 200px;
-
Positioned the image inside the container.
.my-image {
position: absolute;
top: 0;
left: 0;
width: 100%;
This was the important step, because now I could control the image’s size, without affecting its position within the container, which is always at the top.
Finally, I was ready to add the scaling. I could simply adjust the height of the container, and the image would scale down while staying glued to the top.
Here is how I changed the container.
-
Scaled the image by adjusting the container’s height.
.image-container {
height: 150px;
overflow: hidden;
I did set the overflow of the container to hidden to ensure that the scaled-down image wouldn’t spill out of the container. And, we finally did it! The image scaled down, but its top edge stayed right where it was supposed to be.
It took a bit of trial and error, but I finally got it working. It’s one of those things that seems simple on the surface but can get a bit tricky when you dive into the details.